TDD Part 2, Clarifying Software Requirements
Using TDD with Agile Scrum methodology
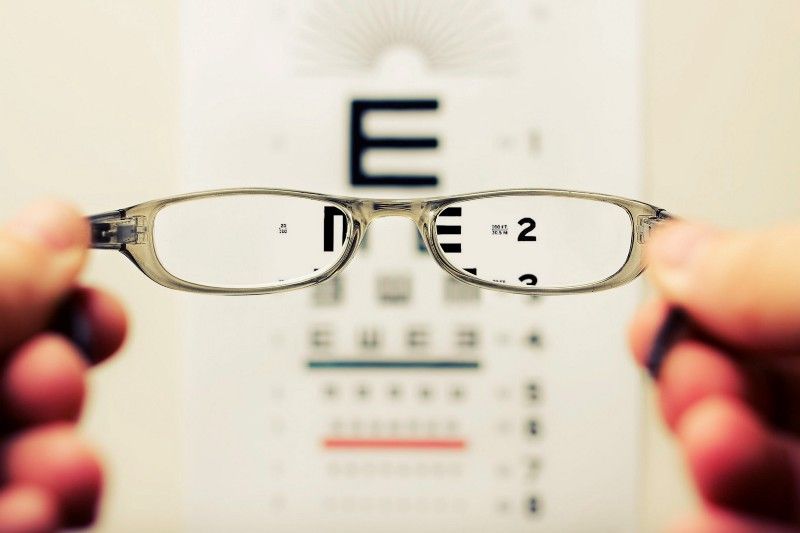
Using TDD with Agile Scrum methodology
High Level to Low Level
After you have scoped your work down to a reasonable amount of work for a sprint, here is a useful way to write user stories, for Jira or whatever you use, for your team to easily understand software requirements.
Start with wireframes
To understand the high level flow of what needs to happen, and what will be displayed, start with wireframes to understand the User’s perspective.
This is useful for engineers working server side as well, so they understand the point of what is being returned per endpoint. This will save time for everyone involved.
How to Phrase User Stories
For the scoped work, phrase each ticket as a User Story as follows:
“As a ____, I want ___, so that ____”
For example: “As a User, I want to be able to sort my list of restaurants by distance closest to me, so that I can walk to the nearest restaurant.”
The first blank is to define the type of User, second blank is the goal of the User, and the third blank is the benefit for the User.
Acceptance Criteria
This is the piece that, in my experience, is the most clarifying. If you do this right, you will have great clarity for what needs to be done for this story to be completed, and exactly what should be tested.
Add it as a description for your User Story with the following phrasing:
“It should ____”
For Example: “It should order the list of restaurants from closest to farthest when the distance filter is checked”
It is typically best to have these listed at a high level for the expected results from a User’s perspective:
“Acceptance Criteria:
- It should order the list of restaurants from closest to farthest when the distance filter is checked
- It should have the original sorted order with the distance filter is unchecked.
- It should have pagination with a maximum of 10 items showing per page.
- It should display the distance of the restaurant at the bottom right corner of the div displaying each restaurant.
- …”
Unit Tests
Often times, it is helpful to start with a function titled closely to the original Acceptance Criteria for the first failing unit test.import { sortByDistance } from './restaurants'describe('sortByDistance()',()=> {
it('should be a function',()=>{
expect(sortByDistance).to.be.a('function'); });
it('should accept an array as an argument',()=>{
}); it('should order the array of restaurants from closest to farthest when the distance filter is checked',()=> {
});});
In typical TDD fashion, this is when you will continue by writing production code to make the test past. Then go back and forth writing your failing test first, then writing the production code to make it pass.
In the example above, the production code would be written in a restaurants.js file in the same directory.
Conclusion
I hope you found this article helpful. It was a super high level overview of how you can clearly write User Stories that easily translate down to Unit Tests for TDD. For a deeper look at this topic, check out this article.